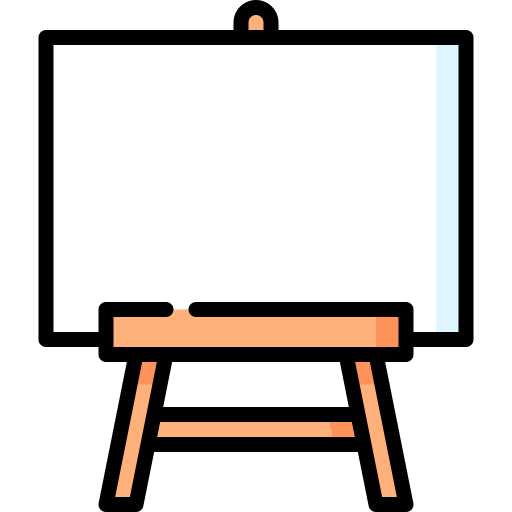
Harnessing the Power of HTML5 and JavaScript: Exploring Canvas
HTML5 and JavaScript provide a powerful combination for creating dynamic and interactive web applications. One of the standout features of HTML5 is the <canvas>
element, which allows developers to draw graphics, animations, and visual effects directly within the browser using JavaScript. In this article, we will delve into the world of canvas and explore how it can be utilized to create stunning visual experiences on the web.
Getting Started with <canvas>
To begin using canvas, simply add the <canvas>
element to your HTML document. This element acts as a container for all the graphics you will draw using JavaScript. It is important to set the width and height attributes to define the desired dimensions of your canvas.
Example:
<canvas id="myCanvas" width="800" height="600"></canvas>
Drawing on the Canvas Once you have set up your canvas, you can use JavaScript to draw various shapes, images, and animations. The <canvas>
element provides a 2D drawing context that you can access using JavaScript methods and properties.
Example:
const canvas = document.getElementById('myCanvas'); const context = canvas.getContext('2d'); // Draw a rectangle context.fillStyle = 'red'; context.fillRect(50, 50, 200, 100); // Draw a circle context.fillStyle = 'blue'; context.beginPath(); context.arc(400, 300, 50, 0, 2 * Math.PI); context.fill();
The code snippet above demonstrates how to draw a rectangle and a circle on the canvas. By accessing the 2D drawing context (context
), you can set properties such as fill color, stroke color, and line width, and then use methods like fillRect()
and arc()
to draw the desired shapes.
Animation and Interactivity Canvas also allows for the creation of dynamic animations and interactive elements. By utilizing JavaScript’s animation techniques, you can update the canvas content at regular intervals to create the illusion of motion.
Example:
function draw() { // Clear the canvas context.clearRect(0, 0, canvas.width, canvas.height); // Draw an animated object context.fillStyle = 'green'; context.fillRect(x, 200, 50, 50); // Update the object's position x += speed; // Check boundaries if (x > canvas.width || x < 0) { speed = -speed; } // Request animation frame requestAnimationFrame(draw); } let x = 0; let speed = 5; draw();
In the above example, an animated object (a green rectangle) moves horizontally across the canvas. The draw()
function is called repeatedly using the requestAnimationFrame()
method to create a smooth animation loop.
Canvas and Beyond
The <canvas>
element offers endless possibilities for creating captivating visual experiences. By combining canvas with other HTML5 features like CSS3 transitions, audio, and video, you can take your projects to new heights.
It’s important to note that canvas requires JavaScript to operate, so consider providing fallback content or alternative experiences for users who have JavaScript disabled or are using assistive technologies.
Conclusion
Canvas is a powerful feature of HTML5 that allows developers to create dynamic, interactive, and visually stunning web experiences. By leveraging JavaScript’s capabilities, you can draw graphics, animations, and visual effects directly within the browser. Explore the possibilities of canvas, experiment with different techniques and styles, and unlock the true potential of HTML5 and JavaScript in your web applications.
No Comments