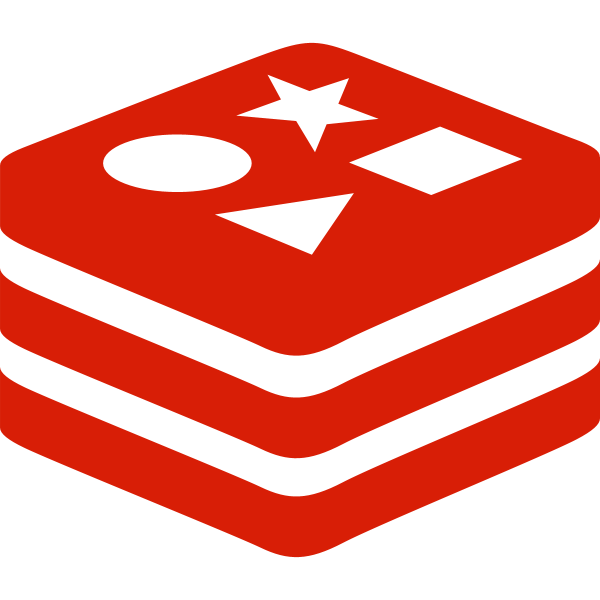
Redis: A Powerful In-Memory Data Store and Cache
Introduction
Redis is an open-source, in-memory data structure store that serves as a high-performance cache and persistent key-value database. It offers a versatile and efficient solution for managing and manipulating data in various applications. In this article, we will explore the features and benefits of Redis, as well as its use cases and advantages over traditional databases.
In-Memory Data Store
One of the key strengths of Redis is its ability to store data in memory, allowing for lightning-fast read and write operations. This makes it ideal for applications that require high-speed data access, such as real-time analytics, caching, and session management. By keeping data in memory, Redis eliminates the disk I/O overhead typically associated with traditional disk-based databases.
Key-Value Data Model
Redis follows a simple key-value data model, where each data entry is associated with a unique key. This simplicity allows for fast retrieval and manipulation of data, as keys can be efficiently indexed and accessed. Redis supports various data types, including strings, lists, sets, sorted sets, and hashes, providing flexibility in storing different types of data.
Caching and Performance Optimization
Redis is commonly used as a cache layer in front of other data sources, such as relational databases or API endpoints. By caching frequently accessed data in Redis, applications can significantly improve response times and reduce the load on backend systems. With its advanced caching features, such as time-to-live (TTL) expiration and automatic eviction policies, Redis ensures efficient memory utilization and optimal cache performance.
Pub/Sub Messaging and Real-Time Data Processing
Redis includes a publish/subscribe messaging system, enabling real-time communication between applications and clients. This pub/sub mechanism allows for the broadcasting of messages to multiple subscribers, making it suitable for building real-time chat applications, event-driven systems, and distributed message queues. Redis also provides features like stream data structures for handling event logs and time-series data.
Scalability and High Availability
Redis supports clustering, which allows for horizontal scaling across multiple nodes, ensuring high performance and availability even under heavy loads. With its distributed architecture and automatic data sharding, Redis can handle large datasets and high request rates. Additionally, Redis provides replication and failover mechanisms, allowing for data redundancy and ensuring continuous operation in case of node failures.
Radis Learning Curve
The learning curve for Redis can vary depending on your prior experience and familiarity with similar technologies. However, Redis is generally considered to have a relatively gentle learning curve compared to other databases or data storage solutions.
An example using Node.js
const redis = require('redis'); // Create a Redis client const client = redis.createClient(); // Set a key-value pair client.set('myKey', 'Hello Redis!', (err, reply) => { if (err) { console.error(err); return; } console.log('Key set:', reply); // Get the value of a key client.get('myKey', (err, value) => { if (err) { console.error(err); return; } console.log('Value:', value); // Delete a key client.del('myKey', (err, reply) => { if (err) { console.error(err); return; } console.log('Key deleted:', reply); // Quit the Redis client client.quit(); }); }); });
In this example, we first create a Redis client using the redis
module. We then set a key-value pair using the set
method, where we set the key 'myKey'
to the value 'Hello Redis!'
. After setting the key, we retrieve its value using the get
method. Finally, we delete the key using the del
method and quit the Redis client.
Please note that to run this code, you’ll need to have the Redis server running locally or provide the necessary connection details if connecting to a remote Redis server. Additionally, make sure you have the redis
module installed by running npm install redis
before running the code.
Conclusion
Redis is a powerful and versatile in-memory data store that offers exceptional performance, flexibility, and scalability. With its ability to act as a cache, persistent key-value store, and real-time messaging system, Redis is widely adopted in various use cases, including web applications, analytics, caching layers, and distributed systems. Its simple yet robust design, coupled with its extensive set of features, makes Redis an invaluable tool for developers looking to optimize performance and enhance data processing capabilities.
No Comments